Are you sure you want to go to an external site to donate a monetary value?
WARNING: Some countries laws may supersede the payment processors policy such as the GDPR and PayPal. While it is highly appreciated to donate, please check with your countries privacy and identity laws regarding privacy of information first. Use at your utmost discretion.
SwitchMenu is obsolete. Please use this one.
The above picture is not visible. The effect is as follows:
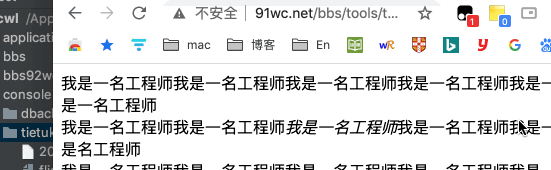
Why forum posts are not allowed to be modified?
The above picture is not visible. The effect is as follows:
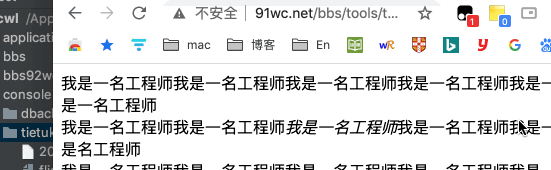
Why forum posts are not allowed to be modified?
@Marti
Sorry, I used to update the library, but I don't know why it became a new library. The new address is here https://openuserjs.org/libs/wishingking/GM_createMenu. I wanted to delete this post, but I don't have the right to delete it. Thank you for your advice! I got it.
When you write a plug-in, you suddenly find that you need a switch button or need to batch add menu.
What should I do?
Call the underlying API to implement?
It may not be too much trouble,
and after deleting the menu, the new menu will change its position up and down.
In short, dig the pit slowly.
Well, there is a switch menu library packaged today,
which perfectly solves the problem of adding switch menu and batch adding menu.
OK, have a good time!
See here for details:
https://openuserjs.org/libs/wishingking/GM_createMenu
You see:

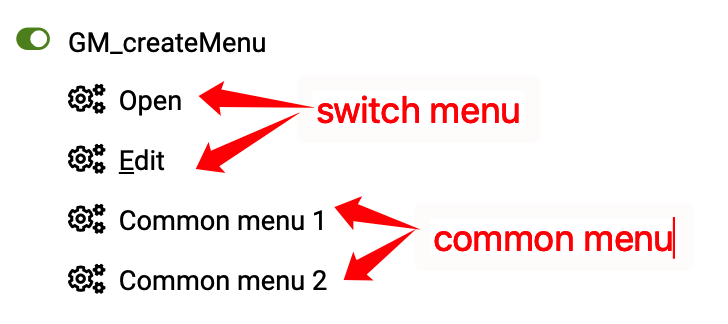
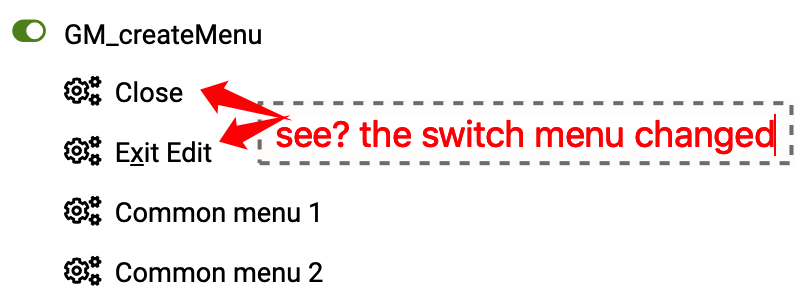
example:
// @grant GM_registerMenuCommand // @grant GM_unregisterMenuCommand // @grant GM_setValue // @grant GM_getValue // @require https://openuserjs.org/src/libs/wishingking/GM_createMenu.js GM_createMenu.add([ //switch menu { on : { default : true, name : "Open", callback : function(){ alert("I'm Open."); } }, off : { name : "Close", callback : function(){ alert("I'm Close."); } } }, { on : { name : "Edit", accessKey: 'E', callback : function(){ alert("I am editing"); } }, off : { default : true, name : "Exit Edit", accessKey: 'X', callback : function(){ alert("I'm exit."); } } }, //common menu { name : "Common menu 1", callback : function(){ alert("I'm Common menu 1"); } }, { name : "Common menu 2", callback : function(){ alert("I am Common menu 2"); } } ]); GM_createMenu.create(); Or: GM_createMenu.add({ on : { default : true, name : "Open", callback : function(){ alert("I'm Open."); } }, off : { name : "Close", callback : function(){ alert("I'm Close."); } } }); GM_createMenu.add({ on : { name : "Edit", accessKey: 'E', callback : function(){ alert("I am editing"); } }, off : { default : true, name : "Exit Edit", accessKey: 'X', callback : function(){ alert("I'm exit."); } } }); GM_createMenu.create();
The last code of the above example is copied incorrectly. The correct code should be as follows:
SwitchMenu.add({ on : { name : "Edit", accessKey: 'E', callback : function(){ alert("I am editing"); } }, off : { default : true, name : "Exit Edit", accessKey: 'X', callback : function(){ alert("I'm exit."); } } });
When you write a plug-in, you suddenly find that you need a switch button.
What should I do?
Call the underlying API to implement?
It may not be too much trouble,
and after deleting the menu, the new menu will change its position up and down.
In short, dig the pit slowly.
Well, there is a switch menu library packaged today,
which perfectly solves the problem of batch adding switch menus.
OK, have a good time!
See here for details:
https://openuserjs.org/libs/wishingking/SwitchMenu
You see:

example:
//GM_registerMenuCommand GM_unregisterMenuCommand must be granted // @grant GM_registerMenuCommand // @grant GM_unregisterMenuCommand // @require https://openuserjs.org/src/libs/wishingking/SwitchMenu.js SwitchMenu.add([ { on : { default : true, name : "Open", callback : function(){ alert("I'm Open."); } }, off : { name : "Close", callback : function(){ alert("I'm Close."); } } }, { on : { name : "Edit", accessKey: 'E', callback : function(){ alert("I am editing"); } }, off : { default : true, name : "Exit Edit", accessKey: 'X', callback : function(){ alert("I'm exit."); } } } ]); SwitchMenu.create(); Or: SwitchMenu.add({ on : { default : true, name : "Open", callback : function(){ alert("I'm Open."); } }, off : { name : "Close", callback : function(){ alert("I'm Close."); } } }); SwitchMenu.add({ on : { default : true, name : "Open", callback : function(){ alert("I'm Open."); } }, off : { name : "Close", callback : function(){ alert("I'm Close."); } } }); SwitchMenu.create();